How To Call An API With Python
APIs for Beginners
TECHNICAL LEARNINGTECH NEWBIESCODING
7/29/20244 min read
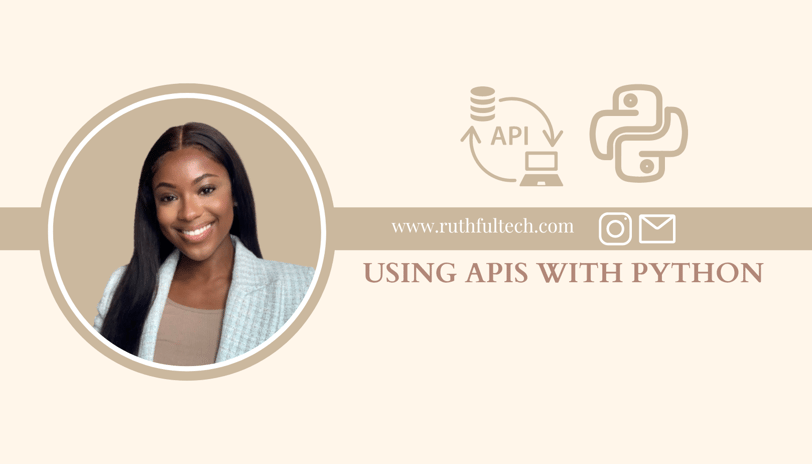
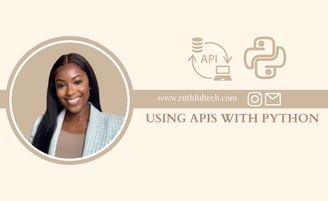
Learning about APIs took my application building to another level. Instead of being limited to static pages or user inputs, I could now present and manipulate all different types of data.
So, what are APIs? It stands for "Application Programming Interface". As a beginner, I didn't think this acronym was very helpful😅, so let's break this down further.
When you order from a menu (your API), you select the meal you want. The waiters take your order and bring you your selected meal (the data in the API world). You don’t have to know or understand how your meal was made; you just send a request and receive it.
Let's look at a real-world example. Instagram has an API that users interact with through the front end. The front end includes features like buttons and search bars, which users can use to request certain data via the API. This data might include the user's follower list or hashtags that other users have used. In the diagram below, when a user clicks the 'followers' button, a request is sent over the internet to an API on a server—a powerful computer that stores and manages data. The server's API queries the database for the follower list, processes the information, and sends it back to the application, where it's displayed to the user.
There are countless APIs available. For instance, you can explore some at API Ninja.
APIs can also interact with each other. For example, a flight comparison website may use APIs from various airlines to find the cheapest flights for you. It can then use another API to provide you with flight times and additional information.
So what requests can you make to an API?
If we look at HTTP requests, you make the following calls:
POST: Create a new resource.
GET: Retrieve data.
PATCH: Apply partial updates to a resource.
PUT: Update or create a resource.
DELETE: Remove a resource.
After sending a request, we will get a response which will include a status code. Have you ever tried clicking on a link and a 404 ‘not found’ error page comes up? This is an example of a status code. They are 3-digit numbers returned by the server and provide info about the success of the request.
Depending on the start of the number, they mean different things. Here are some common response codes:
200 OK: The request was successful.
201 Created: The request was successful and a new resource was created.
400 Bad Request: The server could not understand the request due to invalid syntax.
401 Unauthorized: Authentication is required and has failed or has not been provided.
403 Forbidden: The server understands the request but refuses to authorize it.
404 Not Found: The requested resource could not be found.
500 Internal Server Error: The server encountered an unexpected condition that prevented it from fulfilling the request.
503 Service Unavailable: The server is not ready to handle the request, often due to being overloaded or down for maintenance.
Now For Our Tutorial 💻
Import Requests
This is a Python library that enables us to send HTTP requests. In your terminal, run the command: pip install requests - to install the library using the Python package installer. Add the code 'import requests' onto the top of your Python file
Choose The API You Will Use
Look through the documentation - most APIs will have an API contract which defines how the API is to be used. It outlines the available endpoints and the requests that can be made to these endpoints. Any query parameters, example responses, error handling etc.
Most APIs will require an API key - this is like a password and username combined. It allows the tracing of requested data - ensuring you're not absuing it and makes it easier for access to be revoked in the event. This will be passed through the header as you'll see shortly. Ensure you have followed the steps to generate one.
Make A Call To The Endpoint
In the example below, we have stored our url string in a variable. we use the 'get' method from the requests library we imported and this takes in two arguments, first our API url, and then any headers. In our header, we pass through authorization details which in this case, is our API key.
As an API key should be kept private, I have written 'my_api_key' where your actual one should go.
So we have our response in a JSON format which will contain things like our response code, any response message and our data if received successfully. We want the data in a Python dictionary format, which will make it easier to retrieve the data we want to use in our application. To do this, we use the .json() method.
My data variable holds a JSON structure that looks like this:
{
"item": "Attend Carnaval in Brazil"
}
To retrieve the string of data I want, I will use the bracket notation
In practice, to ensure our code is robust , we need to surround our api with a try and catch block. This is because there is a chance that our api can throw an error when we make a call to it. This may be for several reasons including our server being down, an invalid parameter being passed, authorization issues and so on (see error codes above). We we need a way to catch this and handle it gracefully so any problems can be debugged efficiently.
And there you have it, how to call an API with Python!
If you enjoyed this post, check out these:
How To Convert A Txt File To A PDF Using Python & FPDF
Front-end Development: A Beginner's guide
Top 6 Platforms To Learn How To Code in 2023
And don't forget to subscribe to the bi-weekly newsletter for free resources and tips!
Happy coding!
Ruth
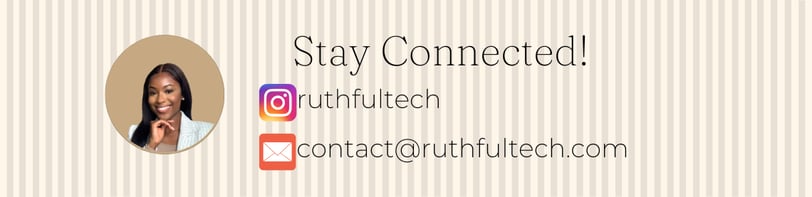
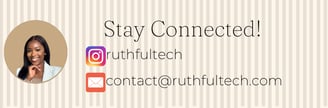